介紹
我們今天要在Unity的Inspector中製作一顆測試按鈕:
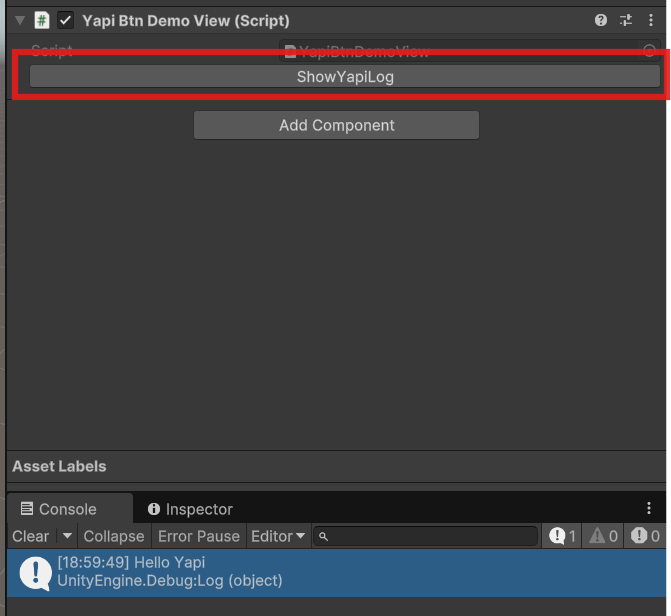
它的特點是你可以直接在Function上掛載Attribute
方便快速進行測試:
[YapiBtn]
public void ShowYapiLog()
{
Debug.Log("Hello Yapi");
}
這個按鈕我們先命名為 - YapiBtn
1. 新增一個Attributes代碼
[AttributeUsage(AttributeTargets.Method)]
public class YapiBtnAttribute : Attribute
{
}
前面的 YapiBtn 名字可以隨意命名
但後面需要加上 Attributes
然後繼承 Attribute 的 Class
接著在上面定義 [AttributeUsage(AttributeTargets.Method)]
表示這個 Attribute 可以被用在 Function 上
其他常見的值還有:
AttributeTargets.Class
:適用於類別。AttributeTargets.Method
:適用於方法。AttributeTargets.Property
:適用於屬性。AttributeTargets.Field
:適用於欄位。AttributeTargets.Constructor
:適用於建構子。AttributeTargets.All
:適用於所有程式元素。AttributeTargets.Class
:適用於類別。AttributeTargets.Method
:適用於方法。AttributeTargets.Property
:適用於屬性。AttributeTargets.Field
:適用於欄位。AttributeTargets.Constructor
:適用於建構子。AttributeTargets.All
:適用於所有程式元素。
2. 創建一個 Yapi Behavior 的代碼
public class YapiBehaviour : MonoBehaviour
{
}
為了不要影響到 MonoBehaviour 的運作
我們可以創建一個自己的 Behaviour 來繼承 MonoBehaviour
3. 新增一個YapiBtnDrawer的Editor代碼
using System;
using System.Reflection;
using UnityEditor;
using UnityEngine;
namespace YapiBtn
{
[CustomEditor(typeof(YapiBehaviour), true)]
public class YapiBtnDrawer : Editor
{
public override void OnInspectorGUI()
{
DrawDefaultInspector();
var targetType = target.GetType();
var methods = targetType.GetMethods(
BindingFlags.Instance |
BindingFlags.Static |
BindingFlags.Public |
BindingFlags.NonPublic);
foreach (var method in methods)
{
if (Attribute.IsDefined(method, typeof(YapiBtnAttribute)))
{
if (GUILayout.Button(method.Name))
{
try
{
if (method.IsStatic)
{
method.Invoke(null, null);
}
else
{
method.Invoke(target, null);
}
}
catch (Exception ex)
{
Debug.LogError($"YapiBtn: [{method.Name}] Execute Error: {ex}");
}
}
}
}
}
}
}
以上的代碼主要是複寫 YapiBehavior 的 Inspector GUI 顯示
我們遍歷所有的 Methods
找到所有有掛載的 YapiBtnAttribute 代碼後
在上面使用 GUILayout.Button 繪製一個按鈕
點擊的時候則呼叫該 Method
4. 新增測試代碼
接下來可以新增一個測試代碼來驗證效果
隨便寫一個 Function, 在上面掛載 [YapiBtn]
public class YapiBtnDemoView : YapiBehavior
{
[YapiBtn]
public void ShowYapiLog()
{
Debug.Log("Hello Yapi");
}
}
然後把它掛載在場景的 GameObject 上
最終效果:
0:00
/0:03
Discussion